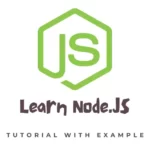
Node.js is a popular JavaScript runtime that allows developers to build fast, scalable, and efficient server-side applications. With its event-driven, non-blocking I/O model, Node.js is ideal for building data-intensive, real-time applications that can handle a large number of simultaneous connections. In this comprehensive Node.js tutorial, we’ll take you through the basics of Node.js and progress to more advanced topics, giving you the knowledge you need to build sophisticated web applications.
What is Node.js?
Node.js is a JavaScript runtime that runs on the server-side, meaning it can execute JavaScript code outside of a web browser. It was developed by Ryan Dahl in 2009 and is built on top of the Google V8 JavaScript engine.
Node.js is designed to be lightweight and efficient, making it ideal for building server-side applications that can handle a large number of simultaneous connections. Node.js is also event-driven, which means it uses callbacks to handle incoming requests, allowing it to handle a large number of requests at once without blocking.
Getting Started
To get started with Node.js, you’ll need to install it on your machine. Node.js is available for Windows, Mac, and Linux operating systems, and you can download it from the official Node.js website.
Once you have Node.js installed, you can create a new Node.js project by opening your terminal or command prompt and typing the following command:
$ mkdir my-node-project
$ cd my-node-project
$ npm init
This will create a new directory called “my-node-project” and initialize a new Node.js project with a package.json file. The package.json file contains information about your project, including its name, version, dependencies, and scripts.
Now that you have a new Node.js project, you can create a new JavaScript file and start writing some code. Let’s create a file called “app.js” and add the following code:
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, world!\n');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
This code creates a new HTTP server that listens on port 3000 and returns the text “Hello, world!” when a client makes a request.
To run this code, save the file and type the following command in your terminal:
$ node app.js
This will start the Node.js server, and you can access it by opening a web browser and navigating to http://localhost:3000/. You should see the message “Hello, world!” displayed in your browser.
Understanding Modules
In Node.js, modules are used to organize code and share functionality between different parts of your application. There are two types of modules in Node.js: built-in modules and external modules.
Built-in modules are modules that come with Node.js and can be used without installing anything extra. For example, the “http” module we used in the previous example is a built-in module that allows us to create HTTP servers.
External modules are modules that are not built into Node.js and need to be installed separately. These modules are typically installed using the Node Package Manager (npm), which is a tool that comes with Node.js and makes it easy to install and manage external modules.
To use an external module, you need to first install it using npm. For example, let’s say we want to use the “express” module to create a web application. We can install the “express” module by typing the following command in our terminal:
$ npm install express
This will download the “express” module and its dependencies and add them to our project’s “node_modules” directory.
Once we’ve installed the “express” module, we can use it in our application by requiring it in our JavaScript code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
This code creates a new "express" application, sets up a route that returns the text "Hello, world!" when a client makes a request to the root URL, and starts the server listening on port 3000.
Asynchronous Programming in Node.js
One of the key features of Node.js is its asynchronous programming model, which allows multiple operations to be executed simultaneously without blocking the event loop. This is achieved using callbacks, promises, and async/await syntax.
Callbacks
Callbacks are functions that are passed as arguments to other functions and are executed when the operation completes. For example, let’s say we want to read a file from disk and return its contents to the client. We can use the built-in “fs” module to read the file, and use a callback to return the contents:
const fs = require('fs');
const http = require('http');
const server = http.createServer((req, res) => {
fs.readFile('file.txt', (err, data) => {
if (err) throw err;
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end(data);
});
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
This code reads the file “file.txt” using the “fs” module, and returns its contents to the client using a callback. Note that the callback is executed asynchronously, which allows the server to continue processing other requests while the file is being read.
Promises
Promises are another way to handle asynchronous operations in Node.js. These are objects that represent the eventual completion (or failure) of an asynchronous operation, and allow you to chain multiple operations together. For example, let’s say we want to read a file from disk, perform some processing on the data, and then return the result to the client. We can use promises to chain these operations together:
const fs = require('fs').promises;
const http = require('http');
const server = http.createServer((req, res) => {
fs.readFile('file.txt')
.then(data => {
const result = process(data);
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end(result);
})
.catch(err => {
console.error(err);
res.writeHead(500, { 'Content-Type': 'text/plain' });
res.end('Internal server error');
});
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
function process(data) {
// Perform some processing on the data
return data.toString().toUpperCase();
}
This code reads the file “file.txt” using the “fs” module’s promises interface, performs some processing on the data using a custom “process” function, and then returns the result to the client. Note that we use the “.then()” method to chain the “readFile” and “process” operations together, and the “.catch()” method to handle any errors that occur.
Async/await syntax
Async/await syntax is a newer way to handle asynchronous operations in Node.js that allows you to write asynchronous code that looks like synchronous code. It is based on promises and is built on top of the same underlying mechanism.
const fs = require('fs').promises;
const http = require('http');
const server = http.createServer(async (req, res) => {
try {
const data = await fs.readFile('file.txt');
const result = process(data);
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end(result);
} catch (err) {
console.error(err);
res.writeHead(500, { 'Content-Type': 'text/plain' });
res.end('Internal server error');
}
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
function process(data) {
// Perform some processing on the data
return data.toString().toUpperCase();
}
This code reads the file “file.txt” using the “fs” module’s promises interface, performs some processing on the data using a custom “process” function, and then returns the result to the client. Note that we use the “async” and “await” keywords to write the asynchronous code that looks like synchronous code, and the “try” and “catch” keywords to handle any errors that occur.
Working with Databases
Node.js is often used for building web applications that interact with databases. There are many database systems that can be used with Node.js, including MySQL, PostgreSQL, MongoDB, and others.
To work with a database in Node.js, we typically use a database driver, which is a module that provides an API for interacting with the database. For example, if we’re using MySQL, we can use the “mysql” module to connect to the database and execute queries:
const mysql = require('mysql2/promise');
const http = require('http');
const connection = await mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'my_database',
});
const server = http.createServer(async (req, res) => {
try {
const [rows, fields] = await connection.execute('SELECT * FROM my_table');
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify(rows));
} catch (err) {
console.error(err);
res.writeHead(500, { 'Content-Type': 'text/plain' });
res.end('Internal server error');
}
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
This code connects to a MySQL database using the “mysql2/promise” module, executes a SELECT query to fetch all rows from a table, and returns the result as a JSON response to the client.
Conclusion
In this Node.js tutorial, we covered the basics of Node.js, including its event-driven architecture, the built-in modules that come with Node.js, and how to use external modules like “express” and “mysql2” to build web applications that interact with databases.
We also covered Node.js’ asynchronous programming model, including how to use callbacks, promises, and async/await syntax to write asynchronous code that doesn’t block the event loop.
By the end of this tutorial, you should have a good understanding of Node.js and how to use it to build web applications. With further practice and learning, you can become a proficient Node.js developer and build robust and scalable web applications using this powerful platform.
We hope you found this Node.js tutorial helpful!