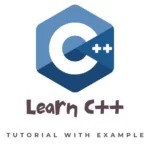
Welcome to our comprehensive basic C++ tutorial! Whether you’re a beginner programmer or an experienced developer looking to refresh your knowledge, this tutorial is perfect for you. In this tutorial, we’ll cover all the essential concepts of C++, including variables, data types, loops, functions, classes and objects, pointers, input/output, exception handling, and the Standard Template Library (STL). By the end of this C++ tutorial, you’ll have a solid foundation in C++ programming and be well on your way to building your own C++ applications or Crack Your C++ Interview. So let’s get started!
Introduction to C++
C++ is a high-performance, general-purpose programming language that was developed by Bjarne Stroustrup in 1979 as an extension of the C Programming Language. It is an object-oriented language, which means that it allows you to define and manipulate data structures called objects
in your code. C++ is a compiled language, which means that the source code is converted into machine code that can be run on a computer.
Setting up a C++ development environment
Before you can start writing C++ code, you’ll need to install a C++ compiler and editor. There are many different options available, such as Visual Studio, Xcode, and Eclipse. Once you have your compiler and editor set up, you’ll need to create a new project and choose a file name for your C++ program.
Basic C++ syntax
C++ has a lot of similarities to C, so if you have previous experience with C, you’ll feel right at home. Here are some basic C++ syntax elements to get you started:
- Variables: Variables are used to store values in C++. You can declare a variable by specifying its type (such as int for integers, float for floating-point numbers, and char for characters) and its name. For example:
int x;
float y;
char c;
- Data types: C++ has a number of built-in data types, including integers, floating-point numbers, characters, and Booleans. You can also create your own custom data types using structs and classes.
- Loops: Loops allow you to execute a block of code multiple times. C++ has three types of loops: for, while, and do-while. Here’s an example of a for loop:
for (int i = 0; i < 10; i++) {
cout << i << endl;
}
- Control structures: Control structures allow you to control the flow of your program. C++ has if-else statements and switch statements. Here’s an example of an if-else statement:
if (x > y) {
cout << "x is greater than y" << endl;
} else {
cout << "x is not greater than y" << endl;
}
Functions
Functions are blocks of code that can be called from other parts of your program. They are useful for organizing your code and making it easier to read and maintain. Here are some key concepts related to functions in C++:
Function declaration: A function declaration tells the compiler about the function’s name, return type, and parameters. It does not provide the implementation of the function.
Function definition: A function definition provides the implementation of the function. It must include the function’s name, return type, parameters, and a block of code.
Function calling: To call a function, you simply use its name followed by a set of parentheses. You can also pass arguments to the function inside the parentheses.
Parameter passing: When calling a function, you can pass values to the function’s parameters. There are two ways to pass parameters in C++: by value and by reference.
Return values: Functions can return a value to the calling code using the return statement. The return type of the function must match the type of the value being returned.
Here’s an example of a simple C++ function that takes two integers as parameters and returns their sum:
int add(int x, int y) {
return x + y;
}
Classes and objects
In C++, a class is a user-defined data type that can contain data and functions. An object is an instance of a class. Classes and objects are key concepts in Object-Oriented Programming (OOP), and they allow you to model real-world concepts in your code.
Here’s an example of a simple C++ class:
class Rectangle {
private:
int width;
int height;
public:
void setWidth(int w) {
width = w;
}
void setHeight(int h) {
height = h;
}
int getArea() {
return width * height;
}
};
To create an object of this class, you can use the following syntax:
Rectangle rect;
You can then access the object’s data and functions using the dot
operator:
rect.setWidth(5);
rect.setHeight(10);
cout << rect.getArea() << endl;
Inheritance is a mechanism by which one class can inherit the properties and methods of another class. This allows you to create a new class that is a modified version of an existing class, without having to rewrite all of the code.
Polymorphism is the ability of a function or object to take on multiple forms. In C++, you can achieve polymorphism using virtual functions and inheritance.
Pointers
Pointers are variables that store the memory address of another variable. They are a key concept in C++ and can be used to manipulate memory and improve the performance of your code.
To declare a pointer in C++, you use the *
operator. For example:
int *ptr;
You can use the &
operator to get the memory address of a variable:
int x = 10;
int *ptr = &x;
You can use the *
operator to access the value stored at a memory address:
int y = *ptr;
Dynamic memory allocation is the process of allocating memory at runtime, rather than at compile time. You can use the new
and delete
operators to dynamically allocate and free memory in C++.
Input/Output
C++ provides a number of ways to read from and write to files and input/output streams. You can use the fstream
library to read and write to files, and the iostream
library to read from and write to standard input/output streams (such as the console).
Here’s an example of reading from a file using the ifstream
class:
#include <fstream>
#include <string>
using namespace std;
int main() {
string line;
ifstream infile("file.txt");
while (getline(infile, line)) {
cout << line << endl;
}
return 0;
}
And here’s an example of writing to a file using the ofstream
class:
#include <fstream>
#include <string>
using namespace std;
int main() {
ofstream outfile("file.txt");
outfile << "Hello, world!" << endl;
return 0;
}
Exception handling
Exception handling is a mechanism for handling runtime errors in C++. It allows you to separate error-handling code from the main logic of your program, making it easier to write and maintain.
To use exception handling in C++, you can use the try
and catch
keywords to enclose the code that may throw an exception, and specify a block of code to handle the exception. Here’s an example:
#include <iostream>
#include <stdexcept>
using namespace std;
int main() {
try {
// code that may throw an exception
throw runtime_error("Something went wrong");
} catch (exception& e) {
// code to handle the exception
cout << e.what() << endl;
}
return 0;
}
You can also throw exceptions using the throw
keyword. This allows you to customize the type and message of the exception being thrown.
Standard Template Library (STL)
The Standard Template Library (STL) is a collection of templates and algorithms that can be used to solve common programming tasks in C++. It includes a number of useful containers, such as vectors, lists, and maps, as well as iterators and algorithms for manipulating and searching through these containers.
Here’s an example of using a vector in C++:
#include <vector>
#include <iostream>
using namespace std;
int main() {
vector<int> v;
v.push_back(10);
v.push_back(20);
v.push_back(30);
cout << v[0] << endl; // prints 10
cout << v[1] << endl; // prints 20
cout << v[2] << endl; // prints 30
return 0;
}
And here’s an example of using an iterator to loop through a vector:
#include <vector>
#include <iostream>
using namespace std;
int main() {
vector<int> v;
v.push_back(10);
v.push_back(20);
v.push_back(30);
for (vector<int>::iterator it = v.begin(); it != v.end(); it++) {
cout << *it << endl;
}
return 0;
}
Advanced C++ features
There are many advanced features in C++ that can help you write more efficient and powerful code. Some of these features include:
- Templates: Templates allow you to write generic code that can work with any data type.
- Namespaces: Namespaces allow you to group related code under a common namespace, to avoid naming conflicts.
- Lambda functions: Lambda functions are anonymous functions that can be used to simplify code.
Here’s an example of a simple template function:
template <typename T>
T max(T x, T y) {
return (x > y) ? x : y;
}
And here’s an example of a lambda function:
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int result = count_if(arr, arr + 5, [](int x) { return x % 2 == 0; });
cout << result << endl; // prints 2
return 0;
}
I hope this C++ tutorial has given you a good foundation in C++ programming. There is much more to learn about C++, but these concepts will get you started on your journey to becoming a proficient C++ developer. Happy coding!