In this article we have gathered the latest and most asked OOPS interview questions and answers for both fresher and experienced. If you’re preparing for a job interview in the field of software development, it’s crucial to have a solid understanding of OOPs concepts and principles. OOPs is a popular programming paradigm used to model real-world objects, systems, and behaviours in code.
Object-Oriented Programming (OOPs) is a popular programming paradigm that emphasizes the use of objects to represent and interact with data and behavior in code. In OOPs, objects are created from classes, which define their properties and methods. OOPs offers several advantages over other programming paradigms, such as encapsulation, inheritance, and polymorphism, which allow for more modular, maintainable, and reusable code. OOPs is widely used in software development and is a fundamental concept for many popular programming languages such as Java, Python, and C++.
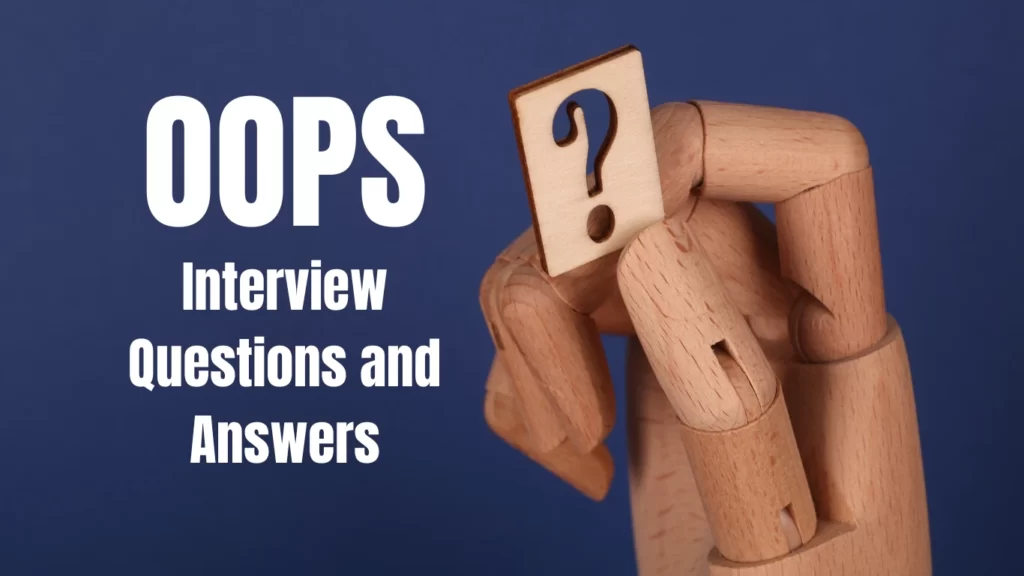
We have prepared this list with short and crisp OOPS interview questions and answers so that it does not take much time to learn. It can also help you to crack the interview to get your dream job.
So let’s get started :
1. What do you mean by OOPs?
2. Name some object-oriented programming languages.
3. What is the purpose of using OOPs concepts?
4. What are the main features of OOPs?
5. Name some advantages of OOPs.
6. What is an object?
7. What is a class?
8. What is Abstraction in OOPs?
9. What are Manipulators?
10. What is an Inline function?
11. What is a virtual function?
12. What is a friend function?
13. What is function overloading?
14. What is operator overloading?
15. What is an abstract class?
16. What is a ternary operator?
17. What is the use of finalize method?
18. What is data abstraction and how can we achieve data abstraction?
19. What is the difference between new and override?
20. What is static and dynamic Binding?
1. What do you mean by OOPs?
OOPs is a programming paradigm used to define objects. The objects can be real-world instances like class having their characteristics and behaviours.
In other words, it is an approach for developing applications that emphasise on objects. An object is a real word entity that contains data and code. It allows binding data and code together.
2. Name some object-oriented programming languages.
There are various OOP languages but the most widely used are:
3. What is the purpose of using OOPs concepts?
OOP aims to implement real world entities like inheritance, hiding, polymorphism in programming. The main purpose of OOP is to bind data and the functions that operate on them together so that no other part of the code can access this data other than that function.
4. What are the main features of OOPs?
The main features of OOPs are :
- Inheritance
- Encapsulation
- Polymorphism
- Data Abstraction
5. Name some advantages of OOPs.
The OOPs have many advantages in programming. Some advantages are
- It follows a bottom-up approach.
- It models the real word well.
- It allows us the reusability of code.
- It promotes the reuse of codes, thus ensuring redundancy.
- OOPs, can create, handle, and maintain complex programming.
- Data Abstraction hides the unnecessary details in OOPs.
- OOPs, work on the bottom-up approach, whereas the structured programming paradigm operates on a top-down approach.
- The OOPs are flexible because of polymorphism.
6. What is an object?
An object contains an instance of the class for all the members. Their behavior is defined in the class template. An object in the real world is an actual entity with which the user interacts. So, the object takes up space and it behaves.
7. What is a class?
A class is a blueprint or template for an object. It is a user-defined data type. Inside a class, we define variables, constants, member functions and other functionality. It does not consume memory at run time. Note that classes are not treated as data structures. It is a logical unit. This is the best example of data binding.
8. What is Abstraction in OOPs?
The first step in writing a program is to define a problem. These problems are derived from real-world issues to which the programmer seeks to find solutions. When it comes to real-world issues it is essential to separate the relevant details from the irrelevant ones. In the process, the programmer comes up with his own abstract model of the problem. This process is called abstraction.
9. What are Manipulators?
Manipulator in OOPs paradigm is simply a function. This function is used on objects in conjunction with the operators such as extraction (>>) and insertion (<<).
For eg. endl and setw.
10. What is an Inline function?
An inline function is an enhancement feature in C++ that is used to increase the program’s execution time.
11. What is a virtual function?
A virtual function is a member function declared within a base class that can be overridden by a derived class. You can call a virtual function for a derived class object when you refer to it using a pointer or a reference to the base class and then perform the function version of the derived class.
Virtual function ensures that the correct function is called for an object regardless of the type of pointer/reference used for the function call. The virtual keyword can be used to implement this function.
12. What is a friend function?
A friend function is a friend of a class that is granted access to the public, private and protected data of that class. It cannot access the data if it is defined outside that class. It can be defined anywhere in the class declaration and access control keywords like public, private or protected cannot affect it.
13. What is function overloading?
Function overloading is a function that enables the programmers to create multiple functions with the same name but different implementation. In other words, these methods with the same name differ from each other by the kind of input and output of the function.
For eg.
- void add(int& a, int& b);
- void add(double& a, double& b);
- void add(struct bob& a, struct bob& b);
14. What is operator overloading?
Operator overloading is also called ad-hoc polymorphism. This is a function where all operators have different implementations depending on the argument type.
Operator overloading is usually defined by the programming language, the programmer, or both.
15. What is an abstract class?
An abstract class is a class which can contain only abstract methods. This class also cannot be instantiated and requires subclasses to give implementation to the abstract methods.
Java programming language allows that only abstract method can exist in an abstract class but other languages allows both abstract and non abstract methods in the abstract class.
16. What is a ternary operator?
In several programming languages, the ternary operator is also called a conditional operator. It is an operator which takes three arguments: the first argument is a comparison argument, the second argument is the result of a true comparison and the last argument is the result of a false comparison. It is like a short way of writing an if-else statement.
17. What is the use of finalize method?
The finalization method is a technique that enables the programmer to clean up resources that are not currently being used. This method is protected and hence accessible only by that particular class or derived class.
18. What is data abstraction and how can we achieve data abstraction?
This is one of the most important features of OOP. It allows us to show only necessary data or information to the user and hides the implementation details from the user. A real-world example of abstraction is driving a car. When we drive a car, we don’t need to know how the engine works (implementation) we only know how the ECG works.
There are two ways to achieve data abstraction
- Abstract class
- Abstract method
19. What is the difference between new and override?
While the NEW modifier tells the compiler to use the new implementation rather than the base class function, OVERRIDE modifier helps to override base class function.
20. What is static and dynamic Binding?
Binding is a simple concept called binding with a class.
Static binding, also known as early binding, is a binding where a name can be associated with a class during compile time.
Dynamic binding, also known as late binding, is a binding where a name can be associated with a class during execution time.
We hope these OOPS Interview Questions help you in cracking your next Programming Interview.
All The Best!