In this article we have gathered the latest and most asked Data Structure Interview Questions and Answers for both freshers and experienced. When it comes to technical interviews, one topic that is sure to come up is data structures. As a job candidate, it’s important to have a solid understanding of the different types of data structures and how they are used in software development.
Computer science and software development make essential use of Data Structures. They organize and store data efficiently, making it easy to access, modify, and analyze. Different types of data structures such as arrays, linked lists, trees, and graphs possess unique characteristics and are chosen for specific situations based on their strengths and weaknesses. Understanding how to use and implement various data structures is a crucial skill for any software developer, as it enables the creation of efficient and effective solutions to complex problems. In this blog post, we’ll discuss some of the most frequently asked data structure interview questions and provide clear and concise answers to help you prepare for your next interview. Whether you’re a beginner or an experienced developer, these data structure interview questions will offer valuable insight into the concepts and terminology you’ll need to know to excel in your next interview.
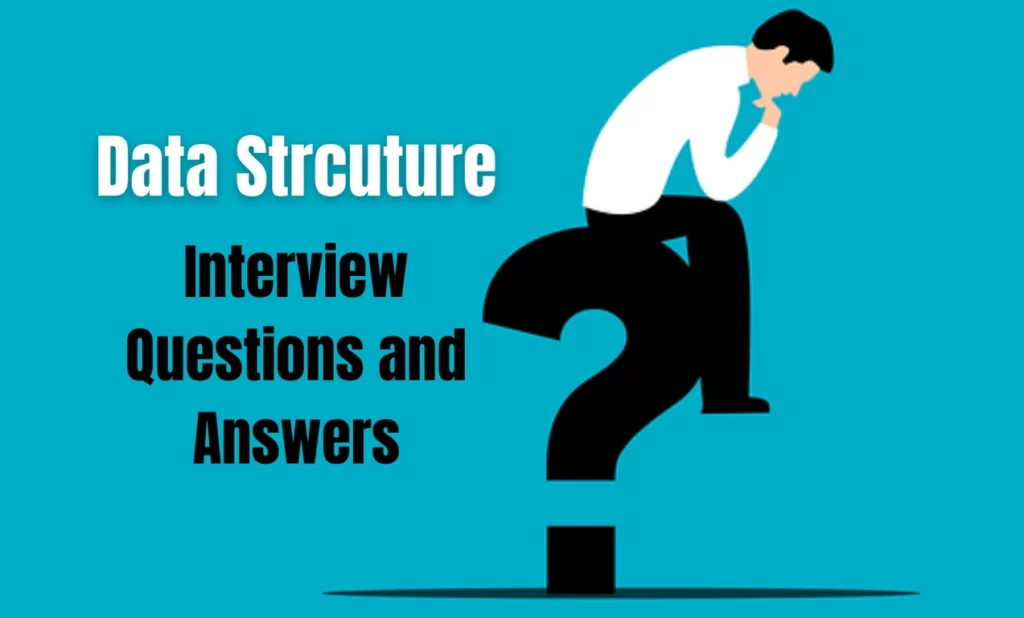
These Data Structure Interview Questions and Answers will help job seekers to prepare well for the job interview and cross the panel discussion easily.
So let’s get started :
1. What is a data structure?
2. What is the difference between an array and a linked list?
3. What is a stack?
4. What is a queue?
5. What is a tree?
6. What is a graph?
7. What is a hash table?
8. What is a heap?
9. What is a trie?
10. What is a Bloom filter?
11. What is a deque?
12. What is a splay tree?
13. What is a AVL tree?
14. What is a Red-Black tree?
15. What is a B-tree?
16. What is a KD-tree?
17. What is a Union-Find data structure?
18. What is a Segment Tree?
19. What is the difference between a stack and a queue?
20. How can you implement a stack using a linked list?
21. How can you reverse a linked list in place?
22. What is a dynamic programming and when it is used?
23. What is a Trie data structure?
24. What is an AVL tree and when it is used?
25. How can you implement Dijkstra’s shortest path algorithm using a priority queue?
1. What is a data structure?
A data structure is a way of organizing and storing data in a computer so that it can be accessed and modified efficiently.
2. What is the difference between an array and a linked list?
An array is a linear data structure that stores elements in contiguous memory locations, while a linked list is a non-linear data structure that stores elements in non-contiguous memory locations, linked together by pointers.
3. What is a stack?
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle, meaning that the last element added to the stack will be the first one to be removed.
4. What is a queue?
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, meaning that the first element added to the queue will be the first one to be removed.
5. What is a tree?
A tree is a non-linear data structure that consists of nodes, where each node has a value and one or more child nodes. The topmost node is called the root, and the nodes that have no children are called leaves.
6. What is a graph?
A graph is a non-linear data structure that consists of nodes (or vertices) and edges that connect the nodes. The edges can be directed or undirected, and the graph can be weighted or unweighted.
7. What is a hash table?
A hash table is a data structure that stores key-value pairs and uses a hash function to map keys to indexes in an array. It is used for efficient lookups, insertions, and deletions.
8. What is a heap?
A heap is a specialized tree-based data structure that satisfies the heap property, which states that the parent node must be greater or smaller than its children, depending on whether it is a max-heap or a min-heap.
9. What is a trie?
A trie (prefix tree) is a tree-based data structure that is used to store a collection of strings. Each node in the trie represents a character in a string, and the path from the root to a leaf node represents a string.
10. What is a Bloom filter?
A Bloom filter is a probabilistic data structure that is used to test whether an element is a member of a set. It has a small amount of false positives but no false negatives, and is efficient in space and time.
11. What is a deque?
A deque (double-ended queue) is a linear data structure that allows for insertion and deletion at both the front and the rear. It can be implemented as a dynamic array or as a doubly-linked list.
12. What is a splay tree?
A splay tree is a self-balancing binary search tree that reorganizes itself after each access to ensure that the recently accessed element becomes the root of the tree. This improves the performance of frequently accessed elements.
13. What is a AVL tree?
An AVL tree is a self-balancing binary search tree that ensures that the difference in the height of left and right subtrees of any node is at most 1. This makes it more balanced than a regular binary search tree and improves the performance of searching and insertion.
14. What is a Red-Black tree?
A Red-Black tree is a type of self-balancing binary search tree that assigns a color (red or black) to each node and enforces certain rules regarding the color of nodes to ensure that the tree remains balanced.
15. What is a B-tree?
A B-tree is a type of self-balancing tree data structure that is primarily used in filesystems and databases for disk-based storage. B-trees are designed to minimize the number of disk seeks by ensuring that all the elements of a subtree are stored in a single block.
16. What is a KD-tree?
A KD-tree is a type of binary search tree that is used to partition a k-dimensional space into smaller subspaces in order to efficiently search for points within a specific range or nearest neighbors.
17. What is a Union-Find data structure?
A Union-Find data structure is a data structure that keeps track of a set of elements partitioned into a number of disjoint (non-overlapping) subsets. It supports two basic operations: finding the subset to which a particular element belongs, and merging two subsets into a single subset.
18. What is a Segment Tree?
A Segment Tree is a data structure that is used to efficiently query for information about an interval or a range within an array. It is a balanced binary tree where each leaf node represents an element of the array and each internal node represents some information about the interval or range represented by its children.
19. What is the difference between a stack and a queue?
A stack follows the Last-In-First-Out (LIFO) principle, meaning that the last element added to the stack will be the first one to be removed. A queue follows the First-In-First-Out (FIFO) principle, meaning that the first element added to the queue will be the first one to be removed.
20. How can you implement a stack using a linked list?
A stack can be implemented using a singly linked list where the top of the stack is the head of the list and the push and pop operations are implemented by adding and removing elements at the head of the list.
21. How can you reverse a linked list in place?
To reverse a linked list in place, you can use a three-pointer approach where you keep track of the current node, the previous node, and the next node. You start with the current node being the head of the list, and in each iteration, you update the pointers so that the previous node becomes the current node and the current node becomes the next node. After each iteration, the current node becomes the head of the list.
22. What is a dynamic programming and when it is used?
Dynamic programming is a technique used to solve problems by breaking them down into smaller subproblems and storing the results of the subproblems to avoid redundant computation. It is used to solve problems that have overlapping subproblems and optimal substructure property.
23. What is a Trie data structure?
A Trie (prefix tree) is a tree-based data structure that is used to store a collection of strings. Each node in the Trie represents a character in a string, and the path from the root to a leaf node represents a string.
24. What is an AVL tree and when it is used?
An AVL tree is a self-balancing binary search tree that ensures that the difference in the height of left and right subtrees of any node is at most 1. This makes it more balanced than a regular binary search tree and improves the performance of searching and insertion. It’s used when we need to maintain a balanced tree and insertion and deletion operations are frequent.
25. How can you implement Dijkstra’s shortest path algorithm using a priority queue?
Dijkstra’s shortest path algorithm can be implemented using a priority queue to efficiently find the vertex with the smallest distance from the starting vertex. The algorithm starts by initializing the distance of the starting vertex to zero and the distances of all other vertices to infinity, and adding all vertices to the priority queue. Then, in each iteration, it extracts the vertex with the smallest distance from the priority queue, and updates the distances of its neighboring vertices if they are smaller than their current distances. This process is repeated until all vertices have been extracted from the priority queue or the target vertex has been extracted.
Data Structures are a fundamental part of computer science and software development. Understanding the different types of data structures and their uses is crucial for any software developer. The questions and answers provided in this blog post should serve as a valuable resource for preparing for technical interviews or for expanding your knowledge of data structures. Remember to brush up on basic concepts such as arrays, linked lists, and trees, as well as more advanced data structures such as hash tables and segment trees. Additionally, be familiar with time and space complexity analysis and common use cases for different data structures. With this knowledge and practice, you will be well-prepared to excel in data structure-related questions in an interview setting.
All The Best!