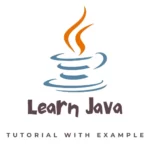
Are you looking to learn Java programming? Look no further! In this Java tutorial, we will cover all the essential concepts you need to know to get started with Java development. From the basics of the Java syntax and data types, to advanced topics like object-oriented programming and exception handling, this tutorial has got you covered. Whether you are a beginner looking to learn programming for the first time, or an experienced developer looking to expand your skill set, this Java tutorial is designed to help you achieve your goals and crack the Java Interview. So grab your favourite code editor and let’s get started!
Introduction to Java and the Java Development Kit (JDK)
Java is a popular programming language that is used for building a wide range of applications, from web and mobile to scientific and financial applications. The Java Development Kit (JDK) is a collection of tools that allows developers to create Java applications.
To get started with Java programming, you will need to install the JDK on your computer. Once you have the JDK installed, you can use a text editor or an Integrated Development Environment (IDE) like Eclipse or IntelliJ to write your Java code.
Basic syntax and data types in Java
In Java, every statement must end with a semicolon (;). Java is a case-sensitive language, which means that variable names and keywords are treated differently based on the capitalization.
Java has a set of primitive data types, such as int (for integers), double (for decimal numbers), and boolean (for true/false values). You can also use variables to store references to objects, which are created using classes.
Here is an example of a Java program that prints “Hello, World!” to the console:
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Operators and expressions in Java
Java has various operators that can be used to perform operations on variables and values. These include arithmetic operators (e.g., +, -, *, /), relational operators (e.g., <, >, ==, !=), and logical operators (e.g., &&, ||, !).
Expressions are combinations of values, variables, and operators that produce a result. For example, the expression “2 + 3” evaluates to 5.
Here is an example of a Java program that uses operators and expressions:
public class Main {
public static void main(String[] args) {
int x = 10;
int y = 5;
int z = x + y;
System.out.println(z); // prints 15
}
}
Control structures in Java
Control structures in Java allow you to control the flow of execution in your program. There are three main types of control structures in Java:
- If-else statements allow you to execute a block of code if a certain condition is true, or a different block of code if the condition is false.
- For loops allow you to execute a block of code multiple times with a loop counter.
- While loops allow you to execute a block of code as long as a certain condition is true.
Here is an example of a Java program that uses an if-else statement:
public class Main {
public static void main(String[] args) {
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
} else {
System.out.println("x is not greater than 5");
}
}
}
Object-Oriented Programming (OOP) concepts in Java
Java is an Object-Oriented Programming language, which means that it is based on the concept of objects. An object is a self-contained unit that contains data and behavior. In Java, objects are created from classes, which define the structure and behaviour of the objects.
There are several key OOP concepts in Java, including:
- Classes: A class is a template that defines the properties and behavior of an object. For example, you could create a class called “Dog” that has properties like “breed” and “name”, and behaviors like “bark” and “fetch”.
- Objects: An object is an instance of a class. You can create multiple objects from a single class, each with its own unique properties and behavior.
- Inheritance: Inheritance allows you to create a new class that is a modified version of an existing class. The new class is called the subclass, and the existing class is the superclass. The subclass can inherit properties and behaviors from the superclass and can also have its own unique properties and behaviors.
- Polymorphism: Polymorphism allows you to create multiple methods with the same name, but with different arguments or return types. This is useful when you want to create a class that can perform similar tasks in different ways.
Here is an example of a Java program that uses OOP concepts:
public class Dog {
// Properties
private String breed;
private String name;
// Constructor
public Dog(String breed, String name) {
this.breed = breed;
this.name = name;
}
// Behaviors
public void bark() {
System.out.println("Woof!");
}
public void fetch() {
System.out.println("Fetching the ball!");
}
// Getters and setters
public String getBreed() {
return breed;
}
public void setBreed(String breed) {
this.breed = breed;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Exception handling in Java
Exception handling in Java allows you to handle runtime errors in your code in a structured way. When an error occurs in your program, it throws an exception, which can be caught and handled by your code. This helps you to prevent your program from crashing and allows you to gracefully handle errors and provide useful feedback to the user.
To handle exceptions in Java, you can use the try-catch block. The try block contains the code that may throw an exception, and the catch block contains the code that will handle the exception.
Here is an example of a Java program that uses exception handling:
public class Main {
public static void main(String[] args) {
try {
int x = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Error: Cannot divide by zero.");
}
}
}
Working with arrays and collections in Java
Arrays in Java allow you to store a fixed-size collection of elements of the same data type. You can access the elements of an array using their index, which starts at 0.
Java also has a number of built-in collections classes that allow you to store and manipulate groups of objects. These include the ArrayList, LinkedList, and HashMap classes, among others.
Here is an example of a Java program that uses an array:
public class Main {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
}
}
And here is an example of a Java program that uses a collection:
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList<String> names = new ArrayList<>();
names.add("John");
names.add("Jane");
names.add("Bob");
for (String name : names) {
System.out.println(name);
}
}
}
Working with files and I/O in Java
The Java Standard Edition (SE) library includes a number of classes that allow you to read and write data to files. You can use the File and FileReader classes to read data from a file, and the FileWriter and PrintWriter classes to write data to a file.
You can also use the Scanner class to read data from the standard input (e.g., the keyboard) or from a file, and the System.out.print and System.out.println methods to write data to the standard output (e.g., the console).
Here is an example of a Java program that reads data from a file:
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
File file = new File("data.txt");
FileReader reader = new FileReader(file);
int c;
while ((c = reader.read()) != -1) {
System.out.print((char) c);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Working with threads in Java
Threads in Java allow you to run multiple pieces of code concurrently in the same program. This is useful when you want to perform tasks in the background or when you want to improve the performance of your program by taking advantage of multiple processors or cores.
To create a thread in Java, you can create a class that extends the Thread class or implements the Runnable interface, and override the run() method. Then, you can create an instance of the thread class and call the start() method to start the thread.
Here is an example of a Java program that uses threads:
public class Main {
public static void main(String[] args) {
Thread thread1 = new Thread(new MyThread("Thread 1"));
Thread thread2 = new Thread(new MyThread("Thread 2"));
thread1.start();
thread2.start();
}
}
class MyThread implements Runnable {
private String name;
public MyThread(String name) {
this.name = name;
}
public void run() {
for (int i = 0; i < 10; i++) {
System.out.println(name + ": " + i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
An introduction to the Java Standard Edition (SE) library and commonly used classes
The Java Standard Edition (SE) library is a collection of classes and interfaces that provide a wide range of functionality for Java developers. Some of the most commonly used classes in the Java SE library include:
- String: The String class represents a sequence of characters and provides methods for manipulating and comparing strings.
- Scanner: The Scanner class allows you to read data from the standard input or from a file.
- Math: The Math class provides a number of mathematical functions and constants, such as trigonometric functions, logarithmic functions, and the value of pi.
- Random: The Random class generates random numbers.
Here is an example of a Java program that uses the String, Scanner, and Math classes:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a string: ");
String input = scanner.nextLine();
System.out.println("The length of the string is: " + input.length());
double x = Math.random();
System.out.println("A random number between 0 and 1: " + x);
}
}
There are many other useful classes in the Java SE library, including classes for working with dates and times (java.util.Date, java.util.Calendar), networking (java.net.), and database connectivity (java.sql.). These and other classes provide a wealth of functionality for building robust and feature-rich Java applications.
These are just a few of the topics that are typically covered in a basic core Java tutorial. By learning these and other fundamental concepts, you can begin to develop your own Java programs and start building your skills as a Java developer. There is much more to learn about Java, including advanced concepts and frameworks, but these basic topics in this Java tutorial will provide a solid foundation for your journey as a Java programmer.