In this article we have gathered the latest and most asked C Programming Interview Questions and Answers for both freshers and experienced. These C interview questions have been designed to familiarise you with the different types of questions you may face during your C programming interview.
C is a middle-level and procedural programming language. Procedural programming language also known as structured programming language is a technique in which large programs are broken down into smaller modules and each module uses structured code. This technique minimises error and misinterpretation.
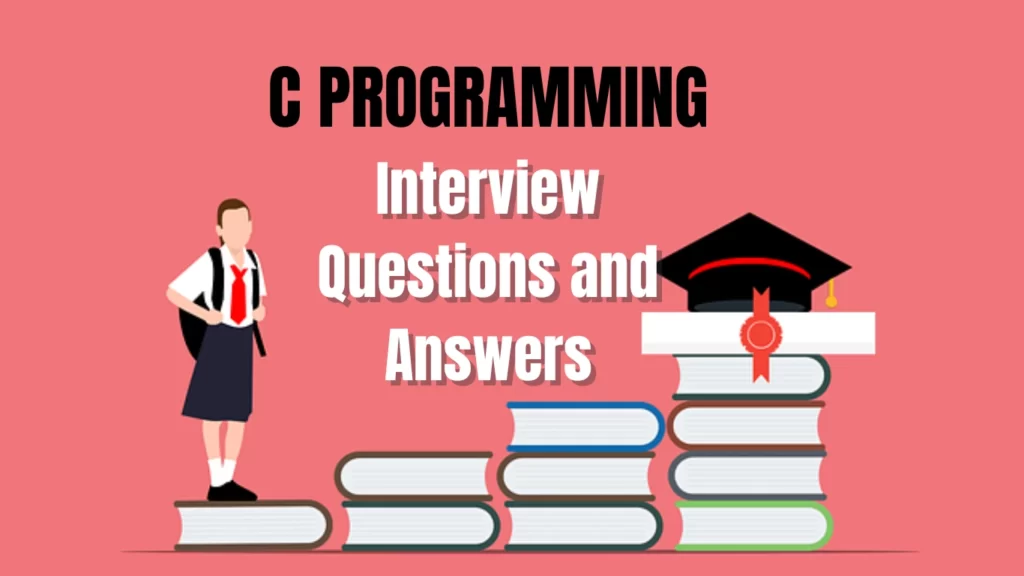
Here are the top frequently asked C Programming Interview Questions and Answers that you must prepare for. These C Programming Interview Questions will help job seekers to prepare well for the job interview and cross the panel discussion easily.
So let’s get started :
1. What is C programming?
2. Why is C known as a mother language?
3. What are the key features of the C Programming language?
4. What do you mean by the pointer in C?
5. What is a stack?
6. What is an array in C?
7. What are header files in C?
8. What is a NULL pointer in C?
9. What is pointer to pointer in C?
10. What is static memory allocation in C?
11. What is dynamic memory allocation in C?
12. What is an auto keyword in C?
13. Can we compile a program without main() function?
14. What is command line argument?
15. What is the maximum length of an identifier?
16. What is typecasting in C?
17. Write a program to print numbers from 1 to 100 without using a loop.
18. Write a program to print “hello world” without using a semicolon.
19. How to write a program in C for swapping two numbers without the use of the third variable?
20. Write a program to check prime number in C Programming?
21. Write a program to check Armstrong number in C?
22. Write a program to reverse a given number in C?
23. What happens if a header file is included twice?
24. What do you mean by a memory leak in C?
25. What is the difference between Source Code and Object Code?
1. What is C programming?
C is one of the oldest programming languages and was developed by Dennis Ritchie in 1972. It is a high-level, general-purpose and structured programming language widely used in various tasks, such as developing system applications, desktop applications, operating systems as well as IoT applications. It is a simple and flexible language used for a variety of scripting system applications, an important part of Windows, Unix and Linux operating systems.
2. Why is C known as a mother language?
C is known as mother language because most of the compilers and JVM are written in C language. Most of the languages that have been developed since C have borrowed heavily from C++, Python, Rust, Javascript, etc. It introduces new core concepts like arrays, functions, file handling which are used in these languages.
3. What are the key features of the C Programming language?
The main features of C language are given below:
- Machine Independent
- Mid-Level programming language
- Memory Management
- Structured programming language
- Simple and efficient
- Function rich libraries
- Case Sensitive
4. What do you mean by the pointer in C?
Pointers are variables in C that store the address of another variable. It allocates memory for variables at run time. The pointer can be of various data types such as int, char, float, double, etc.
5. What is a stack?
Stack is a form of data structure. Data is stored in a stack using the FILO (First In Last Out) approach. On any particular instance, only the top of the stack is accessible, which means that in order to retrieve the data stored inside the stack, the data on the top must first be extracted. Storing data in the stack is also called PUSH, while data retrieval is called POP.
6. What is an array in C?
Array is a group of elements of same type. It has a contiguous memory location. This makes the code optimized, easier to traverse, and easier to sort. The size and type of arrays cannot be changed after its declaration.
There are two types of arrays:
- One-dimensional array: One-dimensional array is an array that stores the elements one after the another.
- Multidimensional array: Multidimensional array is an array that contains more than one array.
7. What are header files in C?
Header files are those that contain C function declarations and macro definitions that are to be shared between sourced files. Header files are generated with the extension .h.
There are two types of header files:
- Programmer generated a header file
- Files that come with your compiler
8. What is a NULL pointer in C?
A pointer which does not refer to any address of value but NULL is known as NULL pointer. When we assign the value ‘0’ to a pointer of any type, it becomes a null pointer.
9. What is pointer to pointer in C?
In case of pointer to pointer concept, a pointer refers to the address of another pointer. Pointer to pointer is an array of pointers. Generally, a pointer contains the address of a variable. Pointer to pointer contains the address of the first pointer. Let us understand this concept through an example:
#include <stdio.h>
int main()
{
int a=10;
int *ptr,**pptr; // *ptr is a pointer and **pptr is a double pointer.
ptr=&a;
pptr=&ptr;
printf("value of a is:%d",a);
printf("\n");
printf("value of *ptr is : %d",*ptr);
printf("\n");
printf("value of **pptr is : %d",**pptr);
return 0;
}
In the above example, pptr is a double pointer that points to the address of the ptr variable and ptr points to the address of the ‘a’ variable.
10. What is static memory allocation in C?
In the case of static memory allocation, the memory is allocated at compile time and the memory cannot be increased during the execution of the program. It is used in array. The lifetime of a variable in static memory is the lifetime of a program. Static memory is allocated using the static keyword. It is implemented using a stack or heap. A pointer is needed to access the variable present in the static memory. Static memory is faster than dynamic memory. Storing variables in static memory requires more memory space.
11. What is dynamic memory allocation in C?
Dynamic memory allocation is the process of memory allocation at run time. There are a set of functions in C used for dynamic memory management i.e. calloc(), malloc(), realloc() and free().
12. What is an auto keyword in C?
In C, each local variable of a function is known as an automatic (auto) variable. Variables that are declared inside a function block are known as local variables. Local variables are also known as auto variables. Using an auto keyword before the data type of the variable is optional. If no value is stored in a local variable, it contains a garbage value.
13. Can we compile a program without main() function?
Yes, we can compile, but it cannot be executed.
But, if we use #define, we can compile and run C program without using main() function. For example:
#include<stdio.h>
#define start main
void start() {
printf("Hello");
}
14. What is command line argument?
The arguments passed to the main() function while executing the program are known as command line arguments. For example:
main(int count, char *args[]){
//code to be executed
}
15. What is the maximum length of an identifier?
It is 32 characters ideally but implementation specific.
16. What is typecasting in C?
Typecasting is a process of converting one data type to another. If we want to store floating type value in int type, we will explicitly convert the data type to another data type.
There are two types of typecasting in C:
- Implicit conversion
- Explicit conversion
Example:
#include <stdio.h>
main() {
int sum = 17, count = 5;
double mean;
mean = (double) sum / count;
printf(“Value of mean : %f\n”, mean );
}
17. Write a program to print numbers from 1 to 100 without using a loop.
Program to print numbers from 1 to 100:
/* Prints numbers from 1 to n */
void printNos(unsigned int n)
{
if(n > 0)
{
printNos(n-1);
printf(“%d “, n);
}
}
18. Write a program to print “hello world” without using a semicolon.
#include<stdio.h>
int main()
{
if(printf("hello world")){}
return 0;
}
19. How to write a program in C for swapping two numbers without the use of the third variable?
#include <stdio.h>
int main()
{
int x, y;
printf(“Input two integers (x & y) to swap\n”);
scanf(“%d%d”, &x, &y);
x = x + y;
y = x – y;
x = x – y;
printf(“x = %d\ny = %d\n”,x,y);
return 0;
}
20. Write a program to check prime number in C Programming?
#include<stdio.h>
#include<conio.h>
void main()
{
int n,i,m=0,flag=0; //declaration of variables.
clrscr(); //It clears the screen.
printf("Enter the number to check prime:");
scanf("%d",&n);
m=n/2;
for(i=2;i<=m;i++)
{
if(n%i==0)
{
printf("Number is not prime");
flag=1;
break; //break keyword used to terminate from the loop.
}
}
if(flag==0)
printf("Number is prime");
getch(); //It reads a character from the keyword.
}
21. Write a program to check Armstrong number in C?
#include<stdio.h>
#include<conio.h>
main()
{
int n,r,sum=0,temp; //declaration of variables.
clrscr(); //It clears the screen.
printf("enter the number=");
scanf("%d",&n);
temp=n;
while(n>0)
{
r=n%10;
sum=sum+(r*r*r);
n=n/10;
}
if(temp==sum)
printf("armstrong number");
else
printf("not armstrong number");
getch(); //It reads a character from the keyword.
}
22. Write a program to reverse a given number in C?
#include<stdio.h>
#include<conio.h>
main()
{
int n, reverse=0, rem; //declaration of variables.
clrscr(); // It clears the screen.
printf("Enter a number: ");
scanf("%d", &n);
while(n!=0)
{
rem=n%10;
reverse=reverse*10+rem;
n/=10;
}
printf("Reversed Number: %d",reverse);
getch(); // It reads a character from the keyword.
}
23. What happens if a header file is included twice?
If a header file is included twice, the compiler will process its contents twice, resulting in an error. You can use guards (#) to prevent a header file from being included multiple times during the compilation process. Thus, even if a header file with the proper syntax is included twice, the second one will be ignored.
24. What do you mean by a memory leak in C?
A memory leak is a type of resource leak that occurs when programmers create memory in the stack and forget to deallocate it. Thus, memory that is no longer needed remains unoccupied. This can also happen when an object is no longer accessible by running code but it is still stored in memory. Memory leaks can result in additional memory usage and can affect the performance of a program.
25. What is the difference between Source Code and Object Code?
The differences between source code and object code are:
Source Code | Object Code |
---|---|
It is created by a programmer. | Object code refers to the output that is generated when the source code is compiled with the C compiler. |
Source code is high-level code. | It is a low-level code. |
Source code is written in plain text by using some high-level programming language like C, C++, Java, or Python. | It is written in machine language. |
It is understandable by humans but not directly understandable by machines. | Object code is machine-understandable but not human-understandable. |
Source code is the input to the compiler or any other translator. | It is the output of the compiler or any other translator. |
We hope these C Programming Interview Questions help you in cracking your next job Interview easily.
All The Best!