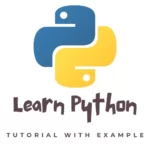
Python is a powerful, high-level programming language that is widely used in the development of web applications, data analysis, artificial intelligence, and scientific computing. It is known for its simplicity, versatility, and large community of developers who contribute to its development and support. If you’re new to programming and want to learn Python, you’re in the right place! In this Python Tutorial, we’ll cover the basics of the Python language and get you up and running with some simple examples.
Before we dive in, it’s important to have a good development environment set up. There are many options for this, but the most popular is the Python IDLE (Integrated Development and Learning Environment). This comes bundled with the Python installation and provides a simple interface for writing and running Python code.
Now let’s get started with some basic concepts in Python.
Variables and Data Types
In Python, we use variables to store and manipulate data. A variable is simply a name that we give to a piece of data, and we can use it to refer to that data in our code.
There are several data types in Python, including integers (whole numbers), floats (decimal numbers), strings (text), and Booleans (True/False values). Here’s how we can create and assign values to variables in Python:
# Assign an integer to a variable
my_var = 5
# Assign a float to a variable
my_float = 3.14
# Assign a string to a variable
my_string = "Hello, world!"
# Assign a Boolean value to a variable
my_bool = True
Operators and Expressions
In Python, we can use various operators to perform operations on variables and data. These include arithmetic operators like +
, -
, *
, and /
for addition, subtraction, multiplication, and division, respectively.
We can also use comparison operators like ==
(equal to), !=
(not equal to), >
(greater than), and <
(less than) to compare values and return a Boolean result.
# Use arithmetic operators
result = 3 + 4
print(result) # Output: 7
# Use comparison operators
is_equal = (3 + 4) == 7
print(is_equal) # Output: True
Control Structures
Control structures allow us to control the flow of our code and make decisions based on certain conditions. In Python, we have several control structures at our disposal, including if
statements, for
loops, and while
loops.
Here’s an example of an if
statement:
x = 5
if x > 0:
print("x is positive")
else:
print("x is not positive")
And here’s an example of a for
loop:
# Print the numbers from 1 to 10
for i in range(1, 11):
print(i)
Functions
Functions are blocks of code that can be defined and then called upon to perform a specific task. In Python, we can define our own functions using the def
keyword. Here’s an example of a simple function that takes an argument and returns a result:
def add_numbers(x, y):
result = x + y
return result
# Call the function and print the result
result =
Lists and Tuples
Lists and tuples are data structures that allow us to store and manipulate collections of items. The Lists are mutable, which means we can add, remove, and modify items in a list. Tuples are immutable, which means we cannot modify the items in a tuple once it is created.
Here’s an example of creating and manipulating a list:
# Create a list
my_list = [1, 2, 3, 4]
# Access an item in the list
print(my_list[0]) # Output: 1
# Modify an item in the list
my_list[0] = 5
print(my_list[0]) # Output: 5
# Add an item to the list
my_list.append(6)
print(my_list) # Output: [5, 2, 3, 4, 6]
# Remove an item from the list
my_list.remove(3)
print(my_list) # Output: [5, 2, 4, 6]
And here’s an example of creating and manipulating a tuple:
# Create a tuple
my_tuple = (1, 2, 3, 4)
# Access an item in the tuple
print(my_tuple[0]) # Output: 1
# Attempt to modify an item in the tuple
# This will cause an error because tuples are immutable
my_tuple[0] = 5
Dictionaries
Dictionaries are another data structure in Python that allow us to store and manipulate collections of items. These are similar to lists, but instead of using integers to access items, we use keys. These keys can be any immutable data type, such as strings or numbers.
Here’s an example of creating and manipulating a dictionary:
# Create a dictionary
my_dict = {'name': 'John', 'age': 25}
# Access an item in the dictionary
print(my_dict['name']) # Output: 'John'
# Modify an item in the dictionary
my_dict['age'] = 26
print(my_dict['age']) # Output: 26
# Add an item to the dictionary
my_dict['city'] = 'New York'
print(my_dict) # Output: {'name': 'John', 'age': 26, 'city': 'New York'}
# Remove an item from the dictionary
del my_dict['age']
print(my_dict) # Output: {'name': 'John', 'city': 'New York'}
Modules and Packages
As you start writing more complex programs in Python, you may find that you need to reuse code that you have written in other projects. Python allows you to organize your code into modules and packages, which can then be imported into other scripts.
Modules are simply Python files that contain code, and packages are collections of modules. To import a module or package, we use the import
keyword.
# Import a module
import math
# Use a function from the module
result = math.sqrt(9)
print(result) # Output: 3.0
# Import a package and access a module within it
import mypackage.mymodule
# Use a function from the module
result = mypackage.mymodule.myfunction(10)
print(result) # Output: 100
Exception Handling
Errors and exceptions are a natural part of programming, and it’s important to handle them properly in your code. In Python, we use try/except blocks to catch and handle exceptions.
Here’s an example of how to use exception handling in Python:
try:
# Some code that may cause an exception
x = 5 / 0
except ZeroDivisionError:
# Handle the exception
print("You can't divide by zero!")
Conclusion
We’ve covered just a few of the basic concepts in Python in this tutorial, but there is much more to learn. Python is a powerful language with a vast ecosystem of libraries and frameworks that can be used to build almost any type of software.
I hope this tutorial has helped you get started with learning Python. If you have any questions or want to learn more, don’t hesitate to reach out!