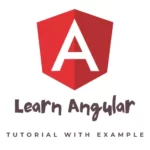
If you’re a beginner looking to get started with Angular, you’ve come to the right place. In this Angular tutorial, we’ll cover everything you need to know to start building web applications using this powerful front-end framework. We’ll walk you through the basics of Angular and provide plenty of examples along the way. By the end of this tutorial, you’ll have a solid understanding of Angular and be able to create your own applications with ease.
What is Angular?
Angular is a popular front-end framework for building web applications. It was developed by Google and is now maintained by a community of developers. Angular is built on top of TypeScript, a superset of JavaScript that adds static typing and other features to the language. Angular provides a set of powerful tools for building web applications, including templates, components, services, and more.
Getting Started with Angular
To get started with Angular, you’ll need to have a basic understanding of HTML, CSS, and JavaScript. You’ll also need to have Node.js and npm (Node Package Manager) installed on your computer. If you haven’t already installed them, you can download them from the official Node.js website.
Once you have Node.js and npm installed, you can install Angular by running the following command in your terminal or command prompt:
npm install -g @angular/cli
This will install the Angular CLI (Command Line Interface), which provides a set of tools for creating, building, and testing Angular applications.
Creating an Angular Application
To create a new Angular application, you can use the Angular CLI. Navigate to the directory where you want to create your application and run the following command:
ng new my-app
This will create a new Angular application with the name “my-app“. The CLI will create a basic project structure and install all the necessary dependencies.
Running the Application
Once your application has been created, you can run it using the Angular CLI. Navigate to the root directory of your application and run the following command:
ng serve
This will start the development server and open your application in your default browser. You should see a basic Angular application with the message “app works!“.
Understanding the Project Structure
Before we dive into building our application, let’s take a look at the project structure created by the Angular CLI:
- src: This directory contains the source code for your application, including the HTML, CSS, and TypeScript files.
- src/app: This directory contains the root component of your application, as well as any additional components you create.
- src/assets: This directory contains any static assets used by your application, such as images or fonts.
- src/environments: This directory contains environment-specific configuration files.
- src/index.html: This is the main HTML file for your application.
- angular.json: This file contains configuration settings for the Angular CLI.
- package.json: This file contains information about your application, including its dependencies.
Creating Components
In Angular, components are the building blocks of your application. Each component encapsulates a specific part of your application and consists of an HTML template, a TypeScript class, and a CSS style sheet. Components are reusable and can be nested within other components.
To create a new component, you can use the Angular CLI. Navigate to the root directory of your application and run the following command:
ng generate component my-component
This will create a new component with the name “my-component” in the “src/app” directory. The CLI will generate the HTML, TypeScript, and CSS files for the component.
In the TypeScript class for your component, you can define the data and functionality for the component. For example, you might define a variable to store data and a method to retrieve or update that data. You can then use interpolation in your HTML template to display that data or event binding to trigger the methods.
Here’s an example of a simple component that displays a message:
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component',
template: '<p>{{ message }}</p>',
})
export class MyComponent {
message = 'Hello, world!';
}
In this example, we’ve defined a component called “MyComponent” with a selector of “app-my-component“. The component has a template that displays the value of the “message” property using interpolation.
Using Components in Templates
Once you’ve created a component, you can use it in your application’s templates by adding its selector to the HTML. For example, if you have a component called “MyComponent“, you can add it to your template like this:
<app-my-component></app-my-component>
When the template is rendered, Angular will replace the “app-my-component” tag with the HTML generated by the “MyComponent” component.
Creating Services
In Angular, services are used to provide data or functionality to your application. Services are typically used to make HTTP requests to external APIs, handle authentication, or provide shared functionality between components.
To create a new service, you can use the Angular CLI. Navigate to the root directory of your application and run the following command:
ng generate service my-service
This will create a new service with the name “my-service” in the “src/app” directory. The CLI will generate a TypeScript file for the service.
In the TypeScript class for your service, you can define the data and functionality for the service. For example, you might define a method to make an HTTP request to an external API and return the response. You can then inject the service into your components and use its methods to retrieve data or perform actions.
Here’s an example of a simple service that makes an HTTP request to an external API:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root',
})
export class MyService {
constructor(private http: HttpClient) {}
getData() {
return this.http.get('https://api.example.com/data');
}
}
In this example, we’ve defined a service called “MyService“. The service uses the “HttpClient” module to make an HTTP GET request to an external API and return the response.
Using Services in Components
Once you’ve created a service, you can use it in your components by injecting it into the constructor. For example, if you have a service called “MyService“, you can inject it into a component like this:
import { Component } from '@angular/core';
import { MyService } from './my-service.service';
@Component({
selector: 'app-my-component',
template: '<p>{{ data }}</p>',
})
export class MyComponent {
data: any;
constructor(private myService: MyService) {}
ngOnInit() {
this.myService.getData().subscribe((data) => {
this.data = data;
});
}
}
In this example, we’ve defined a component called “MyComponent” that injects the “MyService” service. In the “ngOnInit lifecycle” hook, we use the “getData” method of the service to retrieve data from the external API and store it in the component’s “data” property. We use the “subscribe” method to handle the asynchronous response and update the component’s “data” property.
Conclusion
In this Angular tutorial, we’ve covered the basics of building web applications with Angular. We have learned about components and services, two fundamental building blocks of Angular applications.
With components, we can create reusable UI elements that encapsulate their own data and functionality. With services, we can provide data and functionality to our application and share it between components.
Angular is a powerful framework for building web applications. With its extensive set of tools and features, it can help you build modern, responsive, and scalable applications. Whether you’re a beginner or an experienced developer, Angular is a great choice for building web applications. I hope this Angular tutorial has provided you with a solid foundation to get started with this framework.
If you’re interested in learning more about Angular, there are many resources available online, including official documentation, tutorials, and blogs. You can also check out the Angular community on GitHub and Stack Overflow, where you can ask questions and get help from other developers.