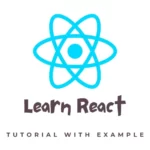
Are you interested in learning how to develop web applications using React? If so, this React tutorial is for you. React is a popular JavaScript library used for building user interfaces, and it is widely adopted by developers worldwide. In this blog post, we will cover React basics, step by step, with examples for beginners. So, let’s get started.
What is React?
React is a JavaScript library for building user interfaces. It was developed by Facebook and is now used by many other companies, including Airbnb, Netflix, and Dropbox. React allows developers to build web apps with reusable UI components, making the development process faster and more efficient.
React is based on the concept of a virtual DOM (Document Object Model), which is a lightweight representation of the actual DOM. The virtual DOM allows React to make changes to the UI without having to reload the entire page. This makes React much faster and more efficient than traditional web development methods.
Getting Started with React
To get started with React, you’ll need to have some basic knowledge of HTML, CSS, and JavaScript. You’ll also need to have Node.js and npm installed on your computer. Once you have these installed, you can create a new React project using the create-react-app tool. Open your terminal and enter the following commands:
npx create-react-app my-app
This will create a new React application in a folder named “my-app“. You can now navigate to the “my-app” folder and start your development server by running the following command:
cd my-app
npm start
This will start your development server, and you can view your React application in your browser at http://localhost:3000.
Creating your first React component
Now that you have set up your development environment let’s create your first React component. A component is a building block of a React application, and it represents a part of your user interface. To create a new component, create a new file called “MyComponent.js” in the “src” folder of your React application. Add the following code to the “MyComponent.js” file:
import React from 'react';
function MyComponent() {
return (
<div>
<h1>Hello, World!</h1>
</div>
);
}
export default MyComponent;
This is a simple React component that displays a heading with the text “Hello, World!“. To use this component in your React application, you need to import it into your App.js file and render it. Add the following code to your “App.js” file:
import React from 'react';
import MyComponent from './MyComponent';
function App() {
return (
<div>
<MyComponent />
</div>
);
}
export default App;
This code imports your “MyComponent” and renders it in the “App” component. If you start your development server, you should see the “Hello, World!” heading in your browser.
Passing props to a React component
Now that you have created your first React component, let’s learn how to pass props to a component. Props are used to pass data from a parent component to a child component. To pass props to your “MyComponent“, you need to modify your “App.js” file. Add the following code to your “App.js” file:
import React from 'react';
import MyComponent from './MyComponent';
function App() {
return (
<div>
<MyComponent name="John" />
</div>
);
}
export default App;
This code passes a prop called “name” with the value “John” to your “MyComponent“. You can access this prop in your “MyComponent” by modifying the “MyComponent.js” file. Add the following code to your “MyComponent.js” file:
import React from 'react';
function MyComponent(props) {
return (
<div>
<h1>Hello, {props.name}!</h1>
</div>
);
}
export default MyComponent;
This code modifies your “MyComponent” to receive props and display the value of the “name” prop in the heading. If you start your development server, you should see the heading with the text “Hello, John!“.
State in React
State is used to manage data that changes over time in a React application. To use state in your React application, you need to modify your “MyComponent” to use state. Add the following code to your “MyComponent.js” file:
import React, { useState } from 'react';
function MyComponent(props) {
const [count, setCount] = useState(0);
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Click me!</button>
</div>
);
}
export default MyComponent;
This code uses the “useState” hook to create a state variable called “count” with an initial value of 0. It also displays the value of the “count” variable in a paragraph and adds a button that increases the value of “count” by one when clicked. If you start your development server, you should see the “Hello, John!” heading, a paragraph with the current value of “count“, and a button that increases the value of “count” when clicked.
Conclusion
In this React tutorial, we have covered React basics, step by step, with examples for beginners. We have learned how to set up a React environment, create a React component, pass props to a component, and use state in a React application. By now, you should have a basic understanding of how React works and how to build simple React applications. If you want to learn more about React, there are plenty of resources available online, including official documentation and tutorials. Good luck with your React journey!